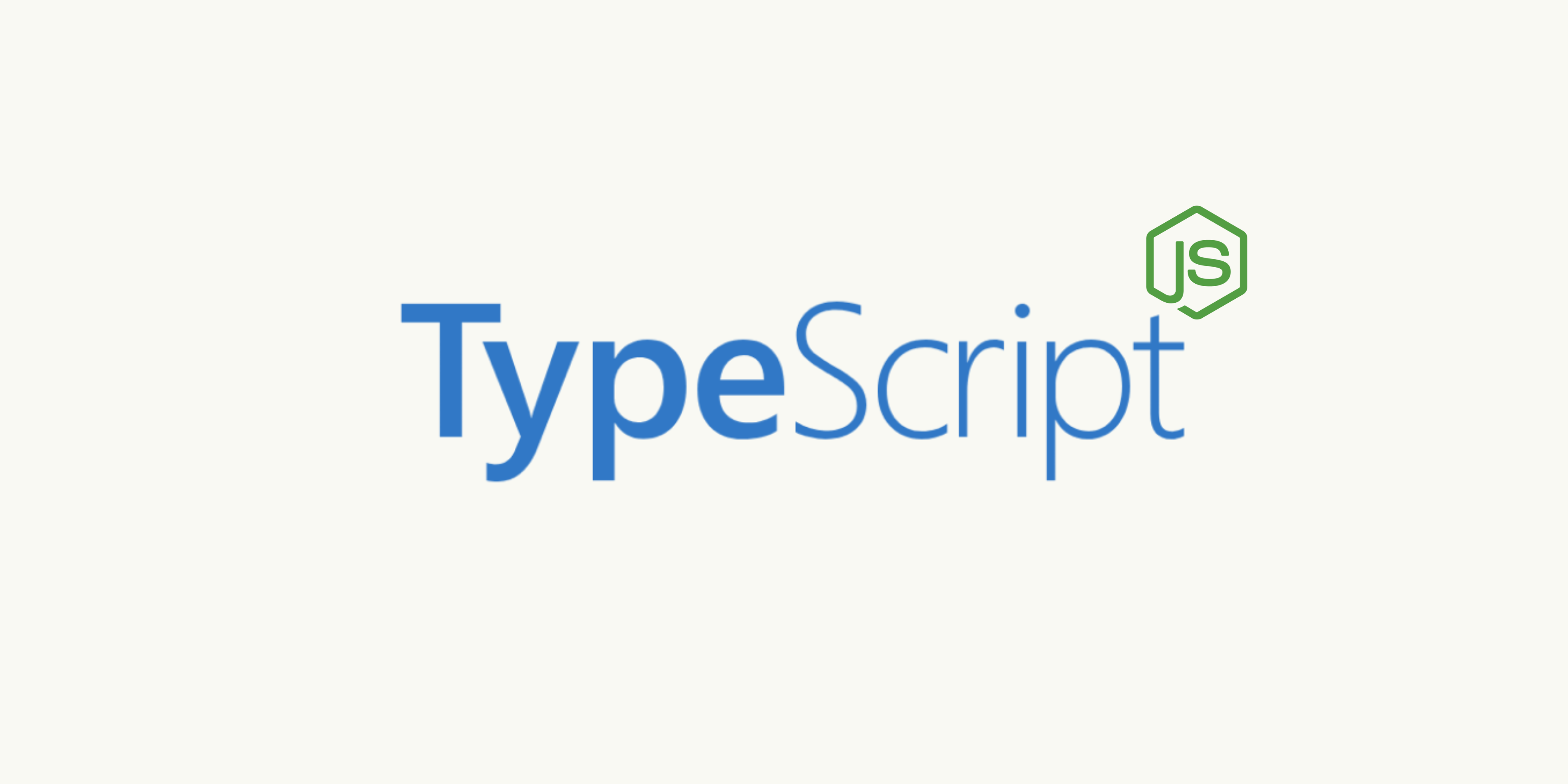
Complete Guide for TS Project
Setting Up a TypeScript Project: A Complete Guide
As a developer working with TypeScript, having a solid project setup is crucial for productive development. In this guide, I’ll walk through creating a TypeScript project from scratch and share some insights I’ve gained along the way.
Initial Setup
First, let’s create our project structure and initialize it:
mkdir my-ts-project
cd my-ts-project
npm init -y
Installing Dependencies
We’ll need TypeScript and some related packages:
npm install typescript --save-dev
npm install @types/node --save-dev
TypeScript Configuration
Generate a TypeScript configuration file:
npx tsc --init
Here’s my recommended tsconfig.json
configuration:
{
"compilerOptions": {
"target": "es2020",
"module": "commonjs",
"lib": ["es2020"],
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"outDir": "./dist",
"rootDir": "./src"
},
"include": ["src/**/*"],
"exclude": ["node_modules"]
}
Project Structure
A clean project structure helps maintain organization:
my-ts-project/
├── package.json
├── tsconfig.json
├── src/
│ └── index.ts
├── dist/
└── node_modules/
Development Workflow
Setting Up Scripts
Add these scripts to your package.json
:
{
"scripts": {
"build": "tsc",
"start": "node dist/index.js",
"dev": "ts-node-dev --respawn src/index.ts"
}
}
Development Environment
For development, we’ll use ts-node-dev
. Here’s why I prefer it:
-
Performance Benefits
- Incremental compilation
- File caching
- Faster restart times
-
Installation
npm install ts-node-dev --save-dev
Alternative Approaches
While I recommend ts-node-dev
, you might also consider:
# Using nodemon with ts-node
npm install nodemon ts-node --save-dev
Then configure in package.json:
{
"scripts": {
"dev": "nodemon --exec ts-node src/index.ts"
}
}
Key Learning Points
-
Why ts-node-dev?
- Better performance through incremental compilation
- Built-in file watching
- TypeScript-specific optimizations
- Memory efficient
-
Common Gotchas
- Remember to include
@types
packages for your dependencies - Keep your
tsconfig.json
updated with your project needs - Be mindful of the
outDir
androotDir
settings
- Remember to include
-
Development Commands
npm run build
- Compiles TypeScript to JavaScriptnpm start
- Runs the compiled JavaScriptnpm run dev
- Runs TypeScript directly with hot reloading
Sample Code
Here’s a basic TypeScript file to get started:
// src/index.ts
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet("TypeScript"));
Conclusion
Setting up a TypeScript project doesn’t have to be complicated. With the right tools and configuration, you can create a development environment that’s both efficient and enjoyable to work with.
Remember that this setup can be customized based on your specific needs. You might want to add:
- ESLint for code linting
- Prettier for code formatting
- Jest or Mocha for testing
- Custom build scripts for production
Keep this guide handy for future reference, and happy coding!